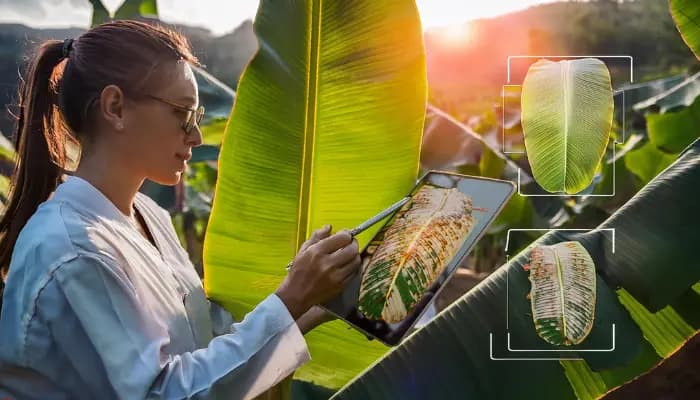
Banana Leaf Disease Detection using Vision Transformer model
Banana cultivation is a significant agricultural activity in many tropical and subtropical regions, providing a vital source of income and nutrition. However, the health and productivity of banana plants are frequently threatened by various leaf diseases. These diseases can drastically reduce yield and quality, leading to substantial economic losses for farmers and impacting food security.
Traditional methods of disease detection in banana plants rely heavily on manual inspection by experts, which can be time-consuming, labor-intensive, and prone to human error. Additionally, by the time symptoms are visually noticeable, the disease may have already spread extensively, making effective management and control more challenging.
To address these challenges, the Banana Leaf Disease Detection project leverages advanced technologies such as machine learning, computer vision, and remote sensing. By utilizing these innovative approaches, the project aims to develop an automated and efficient system for early detection and diagnosis of banana leaf diseases.
Project Overview
Banana Leaf Disease Detection using Vision Transformer and CNN models aims to address a critical agricultural challenge. Banana plants are an essential source of food and income in tropical regions, but diseases can seriously harm their health and productivity. This project uses advanced technology to develop an efficient and accurate system for detecting diseases in banana leaves early.
The combination of Vision Transformer and CNN models offers a powerful approach to solving the problem. Traditional methods of disease detection are time-consuming and labor-intensive. By applying machine learning and computer vision, this project automates disease detection, reducing the reliance on manual inspection. The system helps farmers detect diseases at an early stage, leading to better disease management and increased crop yields.
Prerequisites
Before starting this project, it is important to have a basic understanding of machine learning and computer vision. Familiarity with deep learning frameworks such as TensorFlow and Keras is essential, as they will be used to build the models. A working knowledge of Python programming is required, as it will be used to implement the entire workflow. Experience with data preprocessing, model evaluation, and optimization is also beneficial.
You should have access to a dataset of banana leaf images, labeled with different types of diseases. The dataset will be used to train and validate the models. You will also need access to Google Colab or a similar platform for running the models, as well as the necessary packages such as TensorFlow, Keras, and Sklearn for building and training the models.
Approach
The approach to this project combines advanced machine learning techniques with practical agricultural applications. The project uses a hybrid model, combining the strengths of Vision Transformers and Convolutional Neural Networks (CNNs). Vision Transformers excel at capturing long-range dependencies in image data, while CNNs are powerful for extracting local features. By integrating these two models, the project creates a more robust system for banana leaf disease detection.
The project uses image data of banana leaves, which are preprocessed and fed into both models. Each model predicts the presence of a particular disease, and the final prediction is made using a voting system. This ensures that the predictions are more accurate, as it takes into account the strengths of both models.
Workflow and Methodology
The Overall Workflow of this Project Includes:
- Data Collection and Preparation: The first step involves collecting a dataset of banana leaf images, categorized by the type of disease present. After that, the dataset is prepared for model training.
- Model Building: Two models are built in parallel: a Vision Transformer model and a CNN model. Each model is trained separately to predict banana leaf diseases.
- Model Training: Both models are trained using the prepared dataset. Class weights are adjusted to account for any imbalances in the dataset.
- Model Evaluation: After training, both models are evaluated on a separate validation dataset to check their accuracy.
- Voting Mechanism: The final step involves using an ensemble voting mechanism to combine the predictions of both models and make a more accurate decision.
The Methodology Involves:
- Data Augmentation: Data augmentation techniques are applied to increase the diversity of the training data.
- Model Optimization: Learning rate schedules and early stopping mechanisms are implemented to ensure that the models are optimized during training.
- Performance Evaluation: The models' performance is evaluated using metrics such as precision, recall, accuracy, and AUC (Area Under the Curve).
Data Collection
The success of any machine learning project largely depends on the quality of the data used for training. For this project, a high-quality dataset of banana leaf images is essential. The dataset must include images of healthy banana leaves as well as leaves affected by various diseases like cordana, pestalotiopsis, and sigatoka.
Data Preparation
Once the dataset is collected, it must be carefully prepared before training the models. This involves splitting the dataset into training and validation sets, with 80% of the data used for training and 20% reserved for validation. It is important to ensure that the dataset is balanced so that all disease categories are represented equally.
Data augmentation techniques are used to increase the size of the dataset. These techniques include rotating, flipping, and zooming the images to create new variations. This helps to improve the robustness of the model by exposing it to a wider variety of inputs during training.
Data Preparation Workflow
-
Rescaling: All images are rescaled to a common size of 224x224 pixels to ensure consistency.
-
Normalization: The pixel values of the images are normalized to lie between 0 and 1. This helps the model converge faster during training.
-
Data Augmentation: Techniques such as rotation, zoom, and flipping are applied to increase the size of the dataset.
-
Splitting the Data: The dataset is split into training and validation sets in an 80:20 ratio.
-
Handling Class Imbalance: Class weights are calculated and applied to handle any imbalances in the dataset.
Explanation All Code
Step 1:
Import and install the necessary packages.
!pip install tensorflow
!pip install keras
Importing Libraries
import tensorflow as tf
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, BatchNormalization, MaxPooling2D, Dropout, GlobalAveragePooling2D, Dense, Input, Activation
from tensorflow.keras.preprocessing.image import ImageDataGenerator
from tensorflow.keras.models import Sequential
from tensorflow.keras.layers import Conv2D, MaxPooling2D, Flatten, Dense, Dropout, BatchNormalization, GlobalAveragePooling2D
from tensorflow.keras.applications import ResNet50, InceptionV3
from tensorflow.keras.applications.resnet50 import preprocess_input
from tensorflow.keras.callbacks import EarlyStopping, ModelCheckpoint
from tensorflow.keras.utils import to_categorical
from tensorflow.keras.layers import Dense, LayerNormalization, Dropout, Rescaling
from tensorflow.keras.metrics import Precision, Recall, AUC
from tensorflow.keras.callbacks import LearningRateScheduler
from tensorflow.keras.callbacks import EarlyStopping, ModelCheckpoint
from sklearn.utils import class_weight
from keras.optimizers import Adam
from sklearn.metrics import classification_report, confusion_matrix, precision_score, recall_score, f1_score
import numpy as np
from PIL import Image
from keras.preprocessing.image import img_to_array, load_img
import pandas as pd
import matplotlib.pyplot as plt
import seaborn as sns
from scipy.ndimage import sobel
from skimage.filters import scharr
import warnings
import plotly.express as px
import plotly.subplots as sp
import plotly.graph_objects as go
from plotly.subplots import make_subplots
import plotly.figure_factory as ff
warnings.filterwarnings('ignore')
You can mount your Google Drive in a Google Colab notebook with this piece of code. This makes it easy to view files stored in Google Drive for data manipulation, analysis, and training models in the Colab environment.