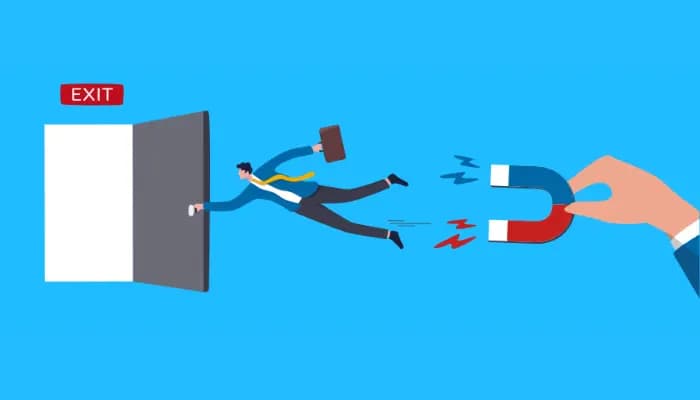
Build a Customer Churn Prediction Model using Decision Trees
Welcome to the customer churn prediction project! Churn prediction helps businesses keep their customers happy and engaged. By using machine learning, we can predict if a customer will leave. In this project, we'll dive into the power of decision trees to build a simple yet effective churn prediction model.
Project Overview
Our objective of this project is to predict customer churn using machine learning techniques. First, we will perform exploratory data analysis of the dataset containing many customer characteristics and prepare it for analysis. The primary algorithm employed is the Decision Tree Classifier, a very proficient algorithm used for classification tasks. To evaluate which of the two approaches is optimal, we employ Logistic Regression as a comparison model
We use SMOTE (Synthetic Minority Over-sampling Technique) also to address the class imbalance problem, which consists of producing samples of the minority classes. The data is then further prepared for the modeling stage by splitting it into training and testing data sets. We assess the model per important standards such as ROC-AUC, confusion matrix, accuracy, precision, recall, and F1-score to make sure that the model is useful and operational in predicting customer churn.
Prerequisites
Learners must develop some skills before undertaking this project Here’s what you should ideally know:
- Understanding of basic knowledge of Python for data analysis and manipulation
- Knowledge of libraries such as Pandas, Numpy, and Matplotlib for data manipulation and data visualization respectively.
- Understanding of data preprocessing steps such as how to deal with missing values, normalization, and scaling.
- Familiarity with exploratory data analysis (EDA) to find out patterns and growing trends in sets.
- Elementary concepts about Decision Tree algorithm to learn how predictive modeling works
- Machine learning frameworks such as Scikit-Learn for building, training, and assessing models
Approach
The initial phase of this customer churn prediction project involves loading and analyzing the dataset to familiarize oneself with its data and figure out any present inconsistencies or missing values. Once the dataset is cleaned by addressing the missing values and encoding the categorical features, we focus on the balancing of the class using SMOTE. The SMOTE technique helps generate synthetic samples of the underrepresented class during classification. After the preprocessing of the data has been done, we proceed to split the data into training and testing datasets to ensure the correctness of the performance evaluation of the model. The Decision Tree Classifier is then fed with the training set to learn the patterns for predicting customer churn while the Logistic Regression model is used for comparative purposes. We assess how well the model resolves the problem using ROC-AUC scores, accuracy, precision, recall and F1 scores. After the results are available, we proceed to optimize the model and modify hyperparameters so that the results can be better.
Workflow and Methodology
Workflow
- Data Collection: Collect the dataset from the public dataset repository, and load it into a DataFrame in Pandas for further analysis.
- Data Cleaning: You need to deal with missing values, convert the categorical data and check that the right data type is used and that all the data is ready for modeling.
- Handling Imbalanced Data: Use SMOTE to generate synthetic samples for the minority class, balancing the dataset.
- Train-Test Split: Split the dataset into training and testing sets to evaluate model performance.
- Model Building: Train a Logistic regression and Decision Tree model using the prepared data.
- Model Evaluation: Evaluate the models using metrics ROC-AUC, confusion matrix, accuracy, precision, recall, and F1-score.
Methodology
The procedure is sequential and commences with an exploration and cleaning of the data. After cleaning the data, there is the application of SMOTE to address the balance of the data set prepared. Afterwards, a Decision Tree Classifier is fitted and its performance is compared with the one achieved using Logistic Regression. Assessment criteria like ROC-AUC, F1, etc. help determine how effective the model is in practice. In the end, the model with the highest accuracy is employed to predict the results for new data.
Data Collection and Preparation
Data Collection:
In this project, we collected the dataset from a public repository. If you are looking to work on a real-world problem, you can get these kinds of datasets from publicly available repositories such as Kaggle, UCI Machine Learning Repository, or company-specific data. We will provide the dataset in this project so that you can work on the same dataset.
Data Preparation Workflow:
- Load the Data: The first step will be to load the dataset into a Pandas DataFrame which can be utilized for analysis.
- Exploratory Data Analysis (EDA): The initial analysis focuses on the data’s structure and its distribution.
- Handle Missing Values: Handle the null values by either filling in or erasing the missing values to achieve an intact dataset.
Code Explanation
STEP 1:
Mounting Google Drive
First, mount Google Drive to access the dataset that is stored in the cloud.
from google.colab import drive
drive.mount('/content/drive')
Installing Necessary Libraries
This piece of code installs very important libraries used in machine and data analysis, notably imbalanced_learn which is used for manipulation of imbalanced data sets, numpy, matplotlib, pandas, and scikit_learn which are used for data processes, graphics, and especially modeling respectively.
!pip install imbalanced_learn
!pip install numpy
!pip install matplotlib
!pip install pandas
!pip install imblearn
!pip install scikit_learn
Importing Required Libraries
This snippet of code imports the libraries that will be required for data analysis, creation, and evaluation of the models. For instance, it involves libraries such as NumPy and Pandas for handling data, Seaborn and Matplotlib for rendering graphs, Scikit-learn and Imbalanced-learn for carrying out machine learning activities including but not limited to regression and classification, feature selection, and working with imbalanced then, and lastly, it includes many of the metrics involved in model evaluation as accuracy_score, roc_auc_score and confusion_matrix, for example.
import warnings
import numpy as np
import pandas as pd
import seaborn as sns
from sklearn import tree
warnings.filterwarnings("ignore")
from matplotlib import pyplot as plt
from sklearn.metrics import roc_curve
from imblearn.over_sampling import SMOTE
from sklearn.feature_selection import RFE
from sklearn.metrics import accuracy_score
from sklearn.metrics import confusion_matrix
from sklearn.tree import DecisionTreeClassifier
from sklearn.preprocessing import OrdinalEncoder
from sklearn.linear_model import LogisticRegression
from sklearn.model_selection import train_test_split
from sklearn.metrics import roc_auc_score,classification_report
from sklearn.metrics import accuracy_score, precision_score, recall_score, f1_score
STEP 2:
Loading Data and Checking Dimensions:
This code loads the CSV file. After loading the dataset it prints the dataset’s shape to check the number of rows and columns. The %time magic command in the notebook records the time taken to perform the task.
Aionlinecourse = pd.read_csv("/content/drive/MyDrive/New 90 Projects/Project_4/data_regression.csv")
%time
print(Aionlinecourse.shape)
Previewing Data
This block of code displays the first few rows of the dataset to have a quick overview of the structure of the dataset.
Aionlinecourse.head()
The purpose of the given code is to provide a summary of the DataFrame Aionlinecourse_housing by displaying the number of records, names of the columns, types of columns, count of non-null values, and the size in memory.
Aionlinecourse.info()
STEP 3:
Data Visualization of Churn Analysis
This code creates a 2x2 grid of visualizations: a distribution of the customer ages, a feature correlation matrix, a count plot for churn by customer gender, and a box plot of the weekly minutes watched for customers who churned and did not churn. It is rearranged to fit the page and for more organization
fig, axes = plt.subplots(2, 2, figsize=(14, 10))
# Distribution of Age
sns.histplot(Aionlinecourse['age'], bins=20, kde=True, ax=axes[0, 0])
axes[0, 0].set_title('Distribution of Customer Age')
axes[0, 0].set_xlabel('Age')
axes[0, 0].set_ylabel('Frequency')
# Correlation Matrix Heatmap
# Select only numerical features for correlation calculation
numerical_df = Aionlinecourse.select_dtypes(include=np.number)
correlation_matrix = numerical_df.corr()
sns.heatmap(correlation_matrix, annot=True, cmap='coolwarm', fmt=".2f", ax=axes[0, 1])
axes[0, 1].set_title('Correlation Matrix of Numerical Features')
# Gender vs Churn
sns.countplot(x='gender', hue='churn', data=Aionlinecourse, ax=axes[1, 0])
axes[1, 0].set_title('Churn Rate by Gender')
axes[1, 0].set_xlabel('Gender')
axes[1, 0].set_ylabel('Count')
# Weekly Minutes Watched vs. Churn
sns.boxplot(x='churn', y='weekly_mins_watched', data=Aionlinecourse, ax=axes[1, 1])
axes[1, 1].set_title('Weekly Minutes Watched vs. Churn')
axes[1, 1].set_xlabel('Churn')
axes[1, 1].set_ylabel('Weekly Minutes Watched')
# Adjust layout to prevent overlap
plt.tight_layout()
plt.show()
Utilizing SMOTE in the Handling of Class Imbalance Datasets
This function prepares the data by incorporating the Synthetic Minority over-sampling technique SMOTE in case of class imbalance. It identifies the numeric features, excludes certain columns, segregates the data into train and test sets, and subsequently employs the SMOTE technique to up-sample the minority class of the train set to achieve equal class distribution.
#Synthetic Minority Oversampling Technique. Generates new instances from existing minority cases that you supply as input.
def prepare_model_smote(df,class_col,cols_to_exclude):
cols=df.select_dtypes(include=np.number).columns.tolist()
X=df[cols]
X = X[X.columns.difference([class_col])]
X = X[X.columns.difference(cols_to_exclude)]
y=df[class_col]
global X_train, X_test, y_train, y_test
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=0)
sm = SMOTE(random_state=0, sampling_strategy=1.0)
X_train, y_train = sm.fit_resample(X_train, y_train)
Clearing Data by Eliminating Missing Values
This code drops the rows that contain null (missing) values to avoid the use of unclear data during the analysis and modeling.
df = Aionlinecourse.dropna() # cleaning up null values
Checking Data Shape
This code will just print the dimensions(Number of rows and columns) of the cleaned df.
print(df.shape)
Previewing Data
This block of code displays the first few rows of the dataset to have a quick overview of the structure of the dataset.
df.head()
Graphical Representation
This script produces a series of plots in the form of a 2x2 grid comprising age distribution in a histogram, a heat map illustrating the correlation of features, a gender-based churn count plot, and a box-plot weekly minutes of watching for churn rate, with some modifications for presentation purposes.
fig, axes = plt.subplots(2, 2, figsize=(14, 10))
# Distribution of Age
sns.histplot(df['age'], bins=20, kde=True, ax=axes[0, 0])
axes[0, 0].set_title('Distribution of Customer Age')
axes[0, 0].set_xlabel('Age')
axes[0, 0].set_ylabel('Frequency')
# Correlation Matrix Heatmap
numerical_df = df.select_dtypes(include=np.number)
correlation_matrix = numerical_df.corr()
sns.heatmap(correlation_matrix, annot=True, cmap='coolwarm', fmt=".2f", ax=axes[0, 1])
axes[0, 1].set_title('Correlation Matrix of Numerical Features')
# Gender vs Churn
sns.countplot(x='gender', hue='churn', data=df, ax=axes[1, 0])
axes[1, 0].set_title('Churn Rate by Gender')
axes[1, 0].set_xlabel('Gender')
axes[1, 0].set_ylabel('Count')
# Weekly Minutes Watched vs. Churn
sns.boxplot(x='churn', y='weekly_mins_watched', data=df, ax=axes[1, 1])
axes[1, 1].set_title('Weekly Minutes Watched vs. Churn')
axes[1, 1].set_xlabel('Churn')
axes[1, 1].set_ylabel('Weekly Minutes Watched')
# Adjust layout to prevent overlap
plt.tight_layout()
plt.show()
STEP 4:
Implementation of SMOTE in Churn Prediction
This code implements SMOTE which is designed to balance the classes in the churn column by performing class oversampling and preparing the dataset into training and testing portions.
prepare_model_smote(df,class_col = 'churn',cols_to_exclude=['customer_id','phone_no', 'year'])
Model Building
This code trains a logistic regression model on the training dataset (X_train, y_train), perform inference on the testing dataset (X_test), and asses the performance of the model via ROC AUC scoring metric. The fitted model (logreg) and the obtained predictions (y_pred) are also declared outside this function and marked as global variables.
#for Logistics Regression
def model_1(X_train,X_test,y_train,y_test):
global logreg #Defines the logistic model as a global model that can be used outside of this function
##Fitting the logistic regression
logreg = LogisticRegression(random_state = 13)
logreg.fit(X_train, y_train)
##Predicting y values
global y_pred #Defines the Y_Pred as a global variable that can be used outside of this function
y_pred = logreg.predict(X_test)
logit_roc_auc = roc_auc_score(y_test, logreg.predict(X_test))
model_1(X_train, X_test, y_train, y_test)
Logistic regression classification report
This block of code generates and outputs a report for the Logistic Regression model as well as various informative metrics such as precision, recall, f1 score, and accuracy for each class of y test compared to predicted y_pred values.
print(classification_report(y_test, y_pred))
Confusion Matrix for Logistic Regression Model
The in-depth analysis of the performance of the Logistic Regression model has been carried out by forming the confusion matrix and exhibiting it in the form of the labeled heatmap for churn and no-churn, also with a brief description for better understanding.
# Create the confusion matrix
cm = confusion_matrix(y_test, y_pred)
# Plot the confusion matrix using seaborn
plt.figure(figsize=(8, 6))
sns.heatmap(cm, annot=True, fmt='d', cmap='Blues',
xticklabels=\['Predicted No Churn', 'Predicted Churn'\],
yticklabels=\['Actual No Churn', 'Actual Churn'\])
plt.title('Confusion Matrix for Logistic Regression')
plt.xlabel('Predicted Label')
plt.ylabel('True Label')
plt.show()
Calculating Model Accuracy
This segment of code measures the accuracy of a Logistic regression model by comparing the predicted labels to the actual test labels and prints the accuracy in percentage form.
# Calculate the accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f"Model Accuracy: {accuracy * 100:.2f}")
Building Decision Tree Model
This piece of code gives an implementation of the function that allows one to fit a decision tree classifier with the use of entropy for making predictions, and then evaluating the model using ROC AUC on the test dataset. The fitted model (dectree) and the predictions (y_pred) have been assigned to global variables so they can be used in other functions.
#Synthetic Minority Oversampling Technique. Generates new instances from existing minority cases that you supply as input
def prepare_model_smote(df,class_col,cols_to_exclude):
cols=df.select_dtypes(include=np.number).columns.tolist()
X=df[cols]
X = X[X.columns.difference([class_col])]
X = X[X.columns.difference(cols_to_exclude)]
y=df[class_col]
global X_train, X_test, y_train, y_test
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.3, random_state=0)
sm = SMOTE(random_state=0, sampling_strategy=1.0)
X_train, y_train = sm.fit_resample(X_train, y_train)
def model_2(X_train,X_test,y_train,y_test):
global dectree #Defines the decision tree model as a global model that can be used outside of this function
##Fitting the decision tree model
dectree = DecisionTreeClassifier(random_state = 13,criterion = 'entropy')
dectree.fit(X_train, y_train)
##Predicting y values
global y_pred #Defines the Y_Pred as a global variable that can be used outside of this function
y_pred = dectree.predict(X_test)
dectree_roc_auc = roc_auc_score(y_test, dectree.predict(X_test))
model_2(X_train, X_test, y_train, y_test)
Decision Tree Classification Report
This block of code generates and outputs a report for the Decision Tree model as well as various metrics informative metrics such as precision, recall, f1 score, and accuracy for each class of y test compared to predicted y_pred values.
print(classification_report(y_test, y_pred))
Confusion Matrix for Decision Tree Model
The in-depth analysis of the performance of the Decision Tree model has been carried out by forming the confusion matrix and exhibiting it in the form of the labeled heatmap for churn and no-churn, also with a brief description for better understanding.
# Create the confusion matrix
cm = confusion_matrix(y_test, y_pred)
# Plot the confusion matrix using seaborn
plt.figure(figsize=(8, 6))
sns.heatmap(cm, annot=True, fmt='d', cmap='Blues',
xticklabels=\['Predicted No Churn', 'Predicted Churn'\],
yticklabels=\['Actual No Churn', 'Actual Churn'\])
plt.title('Confusion Matrix for Decision Tree')
plt.xlabel('Predicted Label')
plt.ylabel('True Label')
plt.show()
Calculating Decision Tree Model Accuracy
This segment of code measures the accuracy of a Decision Tree model by comparing the predicted labels to the actual test labels and prints the accuracy in percentage form.
# Calculate accuracy
accuracy = accuracy_score(y_test, y_pred)
print(f"Model Accuracy: {accuracy * 100:.2f}")
def plot_roc_curve (model,X_test,y_test):
logit_roc_auc = roc_auc_score(y_test, model.predict(X_test))
fpr, tpr, thresholds = roc_curve(y_test, model.predict(X_test))
#Setting the graph area
plt.figure()
plt.xlim([0.0, 1.0])
plt.ylim([0.0, 1.05])
#Plotting the worst line possiple
plt.plot([0, 1], [0, 1],'b--')
#Plotting the logistic regression we have built
plt.plot(fpr, tpr, color='darkorange', label='Model (area = %0.2f)' % logit_roc_auc)
#Adding labels and etc
plt.xlabel('False Positive Rate')
plt.ylabel('True Positive Rate')
plt.title('ROC Curve')
plt.legend(loc="lower right")
plt.savefig('Log_ROC')
plt.show()
STEP 5:
Plotting a ROC Curve
This function enables the plotting of the ROC Curve of the model, which is achieved by computing the False Positive Rate (FPR) and the True Positive Rate (TPR) in the test data and showing the ROC AUC score as well. The straight line bisecting the graph indicates the performance of a model based on chance, whereas the ROC curve for a model in use indicates the discriminant ability of that model.
plot_roc_curve (dectree,X_test,y_test)
Assessment and Comparing of Models
This piece of code implements model performance evaluation of both the Logistic Regression and Decision Tree algorithms in terms of several metrics - accuracy, precision, recall, and F1-measure. It afterward creates a Dataframe for a tabulated representation and comparison of these metrics between the two models.
# Calculate evaluation metrics for both models
def evaluate_model(model, X_test, y_test):
y\_pred = model.predict(X\_test)
accuracy = accuracy\_score(y\_test, y\_pred)
precision = precision\_score(y\_test, y\_pred)
recall = recall\_score(y\_test, y\_pred)
f1 = f1\_score(y\_test, y\_pred)
return accuracy, precision, recall, f1
accuracy_logreg, precision_logreg, recall_logreg, f1_logreg = evaluate_model(logreg, X_test, y_test)
accuracy_dectree, precision_dectree, recall_dectree, f1_dectree = evaluate_model(dectree, X_test, y_test)
# Create a DataFrame to display the results in a table
comparison_df = pd.DataFrame({
'Metric': \['Accuracy', 'Precision', 'Recall', 'F1-Score'\],
'Logistic Regression': \[accuracy\_logreg, precision\_logreg, recall\_logreg, f1\_logreg\],
'Decision Tree': \[accuracy\_dectree, precision\_dectree, recall\_dectree, f1\_dectree\]
})
# Display the table using pandas
display(comparison_df)
Bar Chart for Model Comparison
This section aims to generate a bar plot that compares the two models' performance on metrics such as accuracy, precision, recall, and F1 score among others with proper labeling and legend.
# Create a bar chart to compare model performance
plt.figure(figsize=(10, 6))
bar_width = 0.35
index = np.arange(len(comparison_df['Metric']))
plt.bar(index, comparison_df['Logistic Regression'], bar_width, label='Logistic Regression')
plt.bar(index + bar_width, comparison_df['Decision Tree'], bar_width, label='Decision Tree')
plt.xlabel('Metric')
plt.ylabel('Score')
plt.title('Comparison of Model Performance')
plt.xticks(index + bar_width / 2, comparison_df['Metric'])
plt.legend()
plt.tight_layout()
plt.show()
Visualizing the Decision Tree Model
The purpose of this function is to visualize the Decision Tree model in the graphical representation of the tree as well as features, class names, and the decision threshold. The plot may be tailored by adjusting parameters such as the maximum allowed depth of the tree, the packed structure of the figure, and the text size for optimal visibility.
def plot_model(model,class_names,max_depth=None,figsize=(20,20),fontsize=1):
plt.figure(figsize=figsize)
tree.plot\_tree(dectree
,feature\_names = dectree.feature\_names\_in\_
,fontsize=fontsize
,max\_depth = max\_depth
,class\_names=class\_names
,filled = True);
This code creates a graphical illustration of the instructions employed in the Decision Tree model (dectree) to infer whether or not a customer will churn (churn, not churn).
plot_model(dectree,['not churn','churn'])
This piece of code graphs the Decision Tree (dectree) with a maximum depth of 2 and figure size of (20,20) and with a font size of 10 to enhance the visualization of the decision-making processes for classifying customers as churn or not churn.
plot_model(dectree,['not churn','churn'],max_depth = 2,figsize=(20,20),fontsize=10)
Understanding and Visualizing the Structure of the Decision Tree
The function explores the Decision Tree model (dectree) and reveals the structure of the tree together with its internal elements such as splits and leaves. It shows the details of the nodes and specifies whether the node is a leaf or a split, including the decision rule for split nodes (which feature is being split on, the left node, and the right node).
def read_tree (model):
n_nodes = model.tree_.node_count
children_left = model.tree_.children_left
children_right = model.tree_.children_right
feature = model.tree_.feature
feature_names = model.feature_names_in_
threshold = model.tree_.threshold
node_depth = np.zeros(shape=n_nodes, dtype=np.int64)
is_leaves = np.zeros(shape=n_nodes, dtype=bool)
stack = [(0, 0)] # start with the root node id (0) and its depth (0)
while len(stack) > 0:
\# \`pop\` ensures each node is only visited once
node\_id, depth = stack.pop()
node\_depth\[node\_id\] = depth
\# If the left and right child of a node is not the same we have a split
\# node
is\_split\_node = children\_left\[node\_id\] \!= children\_right\[node\_id\]
\# If a split node, append left and right children and depth to \`stack\`
\# so we can loop through them
if is\_split\_node:
stack.append((children\_left\[node\_id\], depth \+ 1))
stack.append((children\_right\[node\_id\], depth \+ 1))
else:
is\_leaves\[node\_id\] = True
print(
"The binary tree structure has {n} nodes and has "
"the following tree structure:\\n".format(n=n\_nodes)
)
for i in range(n_nodes):
if is\_leaves\[i\]:
print(
"{space}node={node} is a leaf node.".format(
space=node\_depth\[i\] \* "\\t", node=i
)
)
else:
print(
"{space}node={node} is a split node: "
"go to node {left} if {feature} \
"else to node {right}.".format(
space=node\_depth\[i\] \* "\\t",
node=i,
left=children\_left\[i\],
feature=feature\_names\[feature\[i\]\],
threshold=round(threshold\[i\],0),
right=children\_right\[i\],
)
)
read_tree(dectree)
This code shows which features are more important in graphical way to improve the model performance.
def plot_feature_importances(model):
feature_importances = pd.Series(model.feature_importances_, index=model.feature_names_in_)
feature_importances = feature_importances.sort_values(axis=0, ascending=False)
fig, ax = plt.subplots()
feature_importances.plot.bar()
ax.set_title("Feature importances")
fig.tight_layout()
plot_feature_importances(dectree)
Conclusion
The focus of this work was to create a model capable of predicting customer churn with the use of Decision Trees. The first step consisted of the exploration and the cleansing of the data to make sure it was proper for model training. The use of SMOTE helped address class imbalance which in turn enhanced the dataset and improved the model performance. The Decision Tree Classifier showed a much higher ability than Logistic Regression in anticipating customer churn, given that the performance measures of accuracy, precision, recall, and F1-score were all encouraging.
This project illustrates how one can incorporate machine learning techniques in confronting the problem of customer churn — one of the highly worrying issues in the efforts to manage the business. The use of such a model provides an opportunity to devise measures that can be taken to reduce the churn in the user base and enhance the customer experience. The stages of data exploration and preprocessing modeling training and evaluation give the understanding and preconditioning that allows one to build such churn prediction systems in actual business cases.
Challenges New Coders Might Face
Challenge: Handling Missing Data
Solution: Implement imputation methods such as replacing the missing values by mean or median values or more advanced methods such as KNN imputation and K-nearest neighbor imputation should be used.Challenge: Class Imbalance
Solution: Leverage SMOTE (Synthetic Minority Over-sampling Technique) to create synthetic samples of the minority class to balance the data set. This makes sure that the model learns to train the churned customers well.Challenge: Dealing with Categorical Variables
Solution: Incorporate Label Encoding or OneHot Encoding techniques on the categorical variables. Label encoding comes in handy when dealing with ordinal data while one hot encoding is most suitable for categorical features which are not ordinal.Challenge: Feature Selection
Solution: Evaluate the significance of features using the in-built facilities of the model (for example, Decision Tree feature importance) or execute techniques that will ensure only useful features are retained.Challenge: Hyperparameter Tuning for Optimization:
Solution: Use Grid Search or Random Search for hyperparameter tuning to systematically find the optimal settings. These techniques carry out the tuning process automatically, which tends to enhance the performance of the model with very minimal effort.
Frequently Asked Questions (FAQs)
Question 1:What does customer churn prediction mean?
Answer: An effort in customer churn prediction entails the process of trying to ascertain who among the remaining customers of a service or goodwill no longer uses it. It spoils business with losses and helps in taking the right actions to discourage the loss of clients.
Question 2: Why is a Decision Tree used for customer churn prediction?
Answer: Decision Trees are the preferred algorithms for the prediction of customer churn because they are simple to use and interpret, allow both numerical and categorical data, and draw distinct prediction rules that inform of customer churn on critical parameters.
Question 3: What is SMOTE and why is it applied?
Answer: SMOTE is a technique employed to counter the effects of the imbalanced dataset by over-sampling the minority class. In churn prediction, this prediction is often complicated by the fact that the number of customers who churn is usually much lesser than the number of customers who do not churn. SMOTE mitigates this training scenario by synthesizing class samples that lie in the class underrepresented in attempts to train the model.
Question 4: Can this churn prediction model be applied to any business?
Answer: Yes, theoretical models for anticipating churn can be developed in almost any industry such as telecommunication, banking, e-commerce, and services with subscription models. The trick however is to know the right features and modify the model according to what the business requires.
Question 5: How important is feature selection in predicting customer churn?
Answer: Feature selection is an important aspect of churn prediction as it helps to eliminate unnecessary complexity from the data and concentrates on the most useful ones. This increases the accuracy of the model by removing distracting or overlapping features that may otherwise mislead the model.