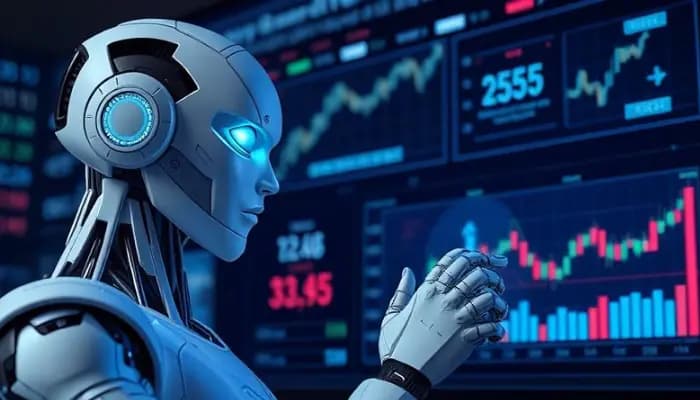
Build ARCH and GARCH Models in Time Series using Python
In this project, we dive into forecasting stock market volatility using ARCH and GARCH models. By analyzing past stock data, we predict future market fluctuations, giving investors and traders a handy tool to make smarter decisions and manage risks with confidence.
Project Overview
This project focuses on analyzing and modeling stock market data using time series methods. The goal is to explore volatility patterns and forecast future market behavior using ARCH and GARCH models. First, the project loads and preprocesses stock price data, including cleaning, handling missing values, and capping outliers. Visualizations like rolling volatility, daily returns distribution, and correlation heatmaps are used to analyze the data. Stationarity tests and seasonal decomposition help in understanding trends and cycles.
Next, the ARCH and GARCH models are fitted to the returns data, and their performance is evaluated based on forecasted volatility. Error metrics like MSE and MAE are computed to compare model accuracy. Finally, the forecasted volatility for both models is plotted to visualize their predictions and assess their performance in forecasting future stock price fluctuations.
Prerequisites
- Python programming knowledge, especially in data analysis and modeling.
- Familiarity with libraries like pandas, numpy, matplotlib, and statsmodels for data manipulation and visualization.
- Understanding of time series analysis, particularly ARCH and GARCH models.
- Basic knowledge of volatility and financial markets.
- Experience with rolling statistics and outlier detection methods.
- Ability to interpret statistical tests like the Augmented Dickey-Fuller (ADF) test for stationarity.
Approach
The project begins by loading stock price data from CSV files and setting up the necessary file paths. The data is then preprocessed by handling missing values, removing irrelevant columns, and ensuring the dataset is clean. Exploratory Data Analysis (EDA) follows, where stock price trends and daily returns are visualized, and rolling volatility is calculated to understand market behavior. The Augmented Dickey-Fuller (ADF) test is applied to check the stationarity of the returns series. Outliers are detected using z-scores and capped based on quantiles to ensure clean data for modeling. Both ARCH and GARCH models are then fitted to the returns data to model volatility, and forecasts for a 5-day horizon are generated. The models' accuracy is evaluated using error metrics like MSE and MAE. Finally, the forecasted volatility from both models is plotted, and their performance is compared to assess which model better captures the market's volatility.
Workflow
- Load the stock price data from CSV files and set the file path.
- Preprocess the data by handling missing values and dropping irrelevant columns.
- Perform exploratory data analysis (EDA) to visualize stock price trends and returns.
- Conduct the Augmented Dickey-Fuller (ADF) test to check for stationarity in the returns.
- Detects and cap outliers using z-scores to clean the dataset.
- Fit ARCH and GARCH models to the returned data.
- Generate volatility forecasts for a 5-day horizon using both models.
- Evaluate model performance using Mean Squared Error (MSE) and Mean Absolute Error (MAE).
- Visualize and compare the forecasted volatility from both models.
Methodology
- Use data preprocessing techniques to ensure the dataset is clean and ready for analysis.
- Apply exploratory data analysis (EDA) to understand key trends and patterns in the stock data.
- Check for stationarity of the returns using the Augmented Dickey-Fuller (ADF) test to ensure valid time series modeling.
- Use z-scores to identify and cap outliers to prevent their influence on model performance.
- Fit both ARCH and GARCH models to the returns series to estimate volatility.
- Forecast future volatility using both models and evaluate the accuracy with MSE and MAE metrics.
- Compare the models' performance and visualize the results to determine which model best forecasts volatility.
Data Collection and Preparation
Data collection
The Time Series dataset is available in Kaggle. It is possible to conveniently and securely access a Kaggle dataset from within Google Colab after configuring your Kaggle credentials to prevent compromising sensitive information. It brings in the user’s data to collect securely the Kaggle API key and username and assigns them as environment variables. This enables the use of Kaggle’s CLI command which authenticates the user and downloads the dataset straight into Colab.
Data preparation workflow
- Load the dataset from the specified file path.
- Check the dataset structure to ensure it’s properly loaded.
- Handle missing values by dropping rows with missing values in key columns like 'Close'.
- Drop irrelevant columns such as 'Trades', 'Deliverable Volume' and '%Deliverble'.
- Ensure the 'Date' column is parsed correctly and set as the index.
- Perform exploratory data analysis (EDA) to understand the data, including visualizations of stock prices and returns.
- Test for stationarity using the Augmented Dickey-Fuller (ADF) test and apply differencing if necessary.
- Detects outliers using z-scores and cap extreme values to maintain data integrity.
- Calculate additional metrics like daily returns and rolling volatility for further analysis.
Understanding the Code
Here’s what is happening under the hood. Let’s go through it step by step:
Step 1:
Mount your Google Drive to access and save datasets, models, and other resources.
from google.colab import drive
drive.mount('/content/drive')
This programming code installs the required Python packages
for performing data analysis and modeling, such as: pandas, numpy, matplotlib, statsmodels, arch, and seaborn.
!pip install pandas numpy matplotlib statsmodels arch seaborn
This code imports libraries for data manipulation, statistical analysis, modeling, and evaluation, including pandas, numpy, statsmodels, and arch.
# Import libraries
import os
import numpy as np
import pandas as pd
import seaborn as sns
from arch import arch_model
from scipy.stats import zscore
import matplotlib.pyplot as plt
from statsmodels.tsa.stattools import adfuller
from statsmodels.tsa.seasonal import seasonal_decompose
from sklearn.metrics import mean_squared_error, mean_absolute_error
This code sets the dataset folder path and checks the contents of the specified directory to verify its structure.
# Dataset folder path
data_folder = '/content/drive/MyDrive/Aionlinecourse_badhon/Project/Build ARCH and GARCH Models in Time Series using Python /nifty50-stock-market-data'
# Check the structure
os.listdir(data_folder)
Step 2:
This code loads the 'ASIANPAINT.csv' dataset into a pandas DataFrame, parsing the 'Date' column as dates and setting it as the index.
data = pd.read_csv(os.path.join(data_folder, 'ASIANPAINT.csv'), parse_dates=['Date'], index_col='Date')
This code displays the first few rows of the dataset to give an overview of its structure and contents.