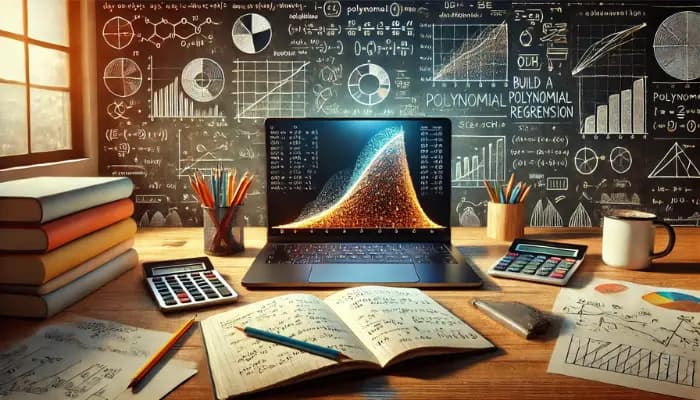
Learn to Build a Polynomial Regression Model from Scratch
Ready to explore polynomial regression? Imagine yourself driving around data - uncovering some hidden structure, some pattern that isn't a simple line. Polynomial regression is like enhancing the power of the model that helps to capture complex curves and trends. Increasing the accuracy for predicting the result and accomplishing actual tasks is such a great idea.
Project Overview
In this project, you will be learning how to create your polynomial regression model from scratch. You will realize how to mold a basic linear model to your advantage. First, let’s collect data and clean it. After that, we will discuss how polynomial regression is different from simple linear regression. Last, we will proceed with coding step by step while explaining it in detail. By the end of this project, you will have a working model that can handle non-linear trends perfectly. Ready to start? Let’s dive in!
Prerequisites
- Basic Python Skills: Be able to write loops, functions, and variables.
- Understanding of Linear Regression: Familiar with drawing a straight line to fit data.
- Basic Math Knowledge: Knowledge in simple algebra, power, and exponentiation.
- Libraries: NumPy and Matplotlib: Familiarity with calculations, data manipulations, and data visualization.
With these basics, you’re ready to start the project!
Approach
Our approach starts by setting up the dataset. After that, we'll use the dataset to train and test our model. We start with a quick review of linear regression to see where polynomial regression can add value. Next, we’ll transform our data by adding polynomial features. This allows our model to capture curves instead of just straight lines. Once the data is prepared, we’ll code the polynomial regression model step-by-step. We will employ the Python programming language and its NumPy library for numerical calculations and transformations. After training, we'll present the results using Matplotlib to assess the data-fitting ability of the model.
In the end, we will do a comparison of these two models and perform a more advanced analysis to show the clear benefit of using this model in practical applications dealing with databases.
Workflow
- Data Collection: First, we gathered the datasets made of the images of healthy and infected leaf samples.
- Data preprocessing: Once we gathered samples, we handled missing values. Then the images were normalized. After that, it was divided into data for training and others for validation.
- Feature Engineering
Add polynomial features to the dataset, which will allow our model to fit curves. - Model Building: Implementing polynomial regression from scratch using Python and NumPy to handle the math.
- Training the Model: The model is then trained on the training dataset to learn from the patterns.
- Evaluation: Carrying out model testing and its performance assessment.
- Comparison with Linear Regression
Compare results with a basic linear regression model to highlight the power of polynomial regression.
Methodology
To construct a polynomial regression model, we start by increasing the dimension of our features by adding polynomial terms of the original features like squares and cubes of the original respectively. This enables the model to fit higher-order trends. We then use least squares estimation to train the model and this is just a concept of getting closer to the real values. By mapping each step, from feature engineering up to training and evaluation, we shall not only know how it works but also find out why the model suits non-linear data. We can visualize and compare the effects of polynomial regression, thus making a practical and useful methodology!
Data Collection
First, we collected a public dataset that shows a non-linear pattern. This will allow us to see the benefits of polynomial regression. You can also use real-world data like housing prices or stock trends. In this dataset, values fluctuate in complex ways.
If you're a beginner, try to create artificial data with a curved pattern. This way at least you will be in control of the data. This makes it easier to know whether the model we are using is functioning as required.
Data Preparation
Once the dataset has been collected, it is then time to prepare the data set. We will start with the missing values so that it doesn’t mess up our data set. Following this, we will normalize our features to ensure all of them are in the same range. This is especially important in polynomial regression. Also, the last step to perform is transforming (squaring, cubing, or taking to higher powers) the original built features to make new features. This transformation enables our model to fit curves rather than mere straight lines. So that more relationships can be captured.
Data Preparation Workflow
- Handle Missing Values: Impute missing values so that it does not reduce the total number of features in a dataset on which the training is going to be done.
- Feature Scaling: Scale features to the same scale for training an accurate model.
- Generate Polynomial Features: To incorporate non-linear relationships, add polynomial features.
- Split the Data: Divide the available data into training and validation data.
Final Check: Check all features for modeling with the conditions that no outliers are influencing the results and no need for scale transformations.
STEP 1:
Code explanation
Here’s what is happening under the hood. Let’s go through it step by step:
Mount Google Drive
Mount your Google Drive to access and save datasets, models, and other resources.
from google.colab import drive
drive.mount('/content/drive')
Suppress Warnings
It excludes non-critical warnings from the output, producing a cleaner view of the results.
import warnings
warnings.filterwarnings('ignore')
Install Required Libraries
This installs the LightGBM and Scikit-Learn libraries. LightGBM is often used for gradient boosting in machine learning, while Scikit-Learn provides essential tools for building and evaluating models.
!pip install lightgbm
!pip install scikit-learn
Import Libraries
This section imports the necessary library for data manipulation (pandas, numpy), graph plotting (seaborn, plotly, matplotlib), statistical operations (scipy), and machine learning models (sklearn).
import sys
import numpy as np
import pandas as pd
import seaborn as sns
import plotly.express as px
import scipy.stats as stats
import matplotlib.pyplot as plt
from sklearn import linear_model
import plotly.graph_objects as go
from plotly.subplots import make_subplots
from sklearn.experimental import enable_iterative_imputer
from sklearn.impute import IterativeImputer
from sklearn.preprocessing import PolynomialFeatures
from sklearn.impute import KNNImputer
from sklearn.impute import SimpleImputer
from sklearn.model_selection import train_test_split
STEP 2:
Load the Dataset
The code employs the pandas library to retrieve the dataset from Google Drive. The head() method shows the first few records within the dataset to have an overview of the dataset.