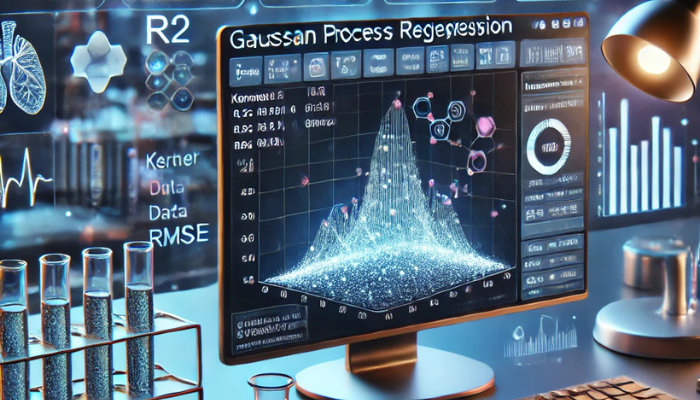
Time Series Analysis and Prediction of Healthcare Trends Using Gaussian Process Regression
Explore the intriguing domain of Gaussian Process Regression-based Healthcare trend prediction! This project merges state-of-the-art machine learning algorithms with the time-series analysis of industry data. Simplifying the complex steps makes this guide easy to follow and effective in learning about predictive modeling without being boring.
Project Overview
This project explores the trends of the Healthcare industry using Gaussian Process Regression which is very useful in machine learning. This process comprises several stages beginning with data loading followed by data preprocessing, where timestamps and frequencies are set to enable time-series data. Patterns of the data are then visualized to analyze how they relate to trends in the data and for recognition of inherent features.
Since the data is non-stationary, different methods are used to prepare the data set for modeling. In this regard, a kernel is defined regarding the Gaussian process modeling period to address the varying periodic and nonlinear trends present in the data. The model is built on different data and performance metrics such as R², MAE and RMSE. Which helps to ensure the model’s efficiency is used in the model evaluation. The results of the forecasts are plotted with the confidence intervals indicating the range which has a risk of variation.
One of the project's most important elements is how the predictions are transformed back to the original scale at the end after differencing has been done to make the findings relevant. This allows the reader to appreciate the considerations for residual analysis and error estimation better and allows this project to solve practical issues in time-series forecasting. It is the ideal combination of data science and machine learning for people who consider themselves ready for a different type of challenge!
Prerequisites
Before commencing this project, ensure that you have the following skills and tools:
- Familiarity with Python and the libraries Pandas, NumPy, and Matplotlib.
- Knowledge of machine learning including regression models and time series analysis.
- Familiarity with Gaussian Processes and kernels
Approach
The process starts with the loading and preprocessing of the data in which timestamps are created and time series forecasting is done with a monthly frequency for the compatibility of time series. The next step is to analyze the trends embedded in the Healthcare data through the application of line and density plots in the search and identification of patterns and distributions. To solve the problem of non-stationarity, the time series data is different, given that the time series modeling is based entirely on the assumption of constant mean and variance. Then, a kernel is built for Gaussian Process Regressor which accounts for both periodicity and non-linear factors. The model is fit on the differenced data, and forecasts are made for both within-sample and out-of-sample data, including prediction intervals for the forecasts. Lastly, the differences are scaled back to the actual scale for the predictions, allowing proper assessment of the actual values. In the entire process, other metrics such as R², MAE and RMSE, as well as residual analysis, are also computed and done to assess the performance of the model.
Workflow and Methodology
- Data Loading: Load the dataset available at the given path for analysis.
- Data Preprocessing: Transform time to time, determine the seasonality to be months, and arrange the data for time series analysis.
- Data Visualization: Draw density plots and QQ plots to examine the relationships and character of data.
- Stationarity Handling: Apply the differencing methods to make the data stationary so that the model fits well.
- Model Design: Create a specialized Gaussian Process kernel that can capture the periodic and non-linear behavior present in the data.
- Model Training: Fit the Gaussian Process Regressor to the training dataset that has been processed for this purpose.
- Predictions and Uncertainty: Generate the predictions for both training and test sets along with appropriate confidence distribution to indicate uncertainty.
- Reverting Differenced Data: Carry out inverse transformation for the prediction to enable effective comparison of the predictions and the actual data.
- Model Evaluation: Determine the effectiveness of the model using evaluation approaches like R², MAE and RMSE, also do residuals analysis.
Data Collection and Preparation
Data Collection:
In this project, we collected the dataset from a public repository. If you are looking to work on a real-world problem, you can get these kinds of datasets from publicly available repositories such as Kaggle, UCI Machine Learning Repository, or company-specific data. We will provide the dataset in this project so that you can work on the same dataset.
Data Preparation Workflow:
- Import the dataset into DataFrame, changing the month column to timestamp.
- Set the index as the month column and make sure to have the monthly frequency.
- For non-stationary data, apply differencing.
- Modeling would then split the prepared data into the training and test sets.
Code Explanation
STEP 1:
Mounting Google Drive
First, mount Google Drive to access the dataset that is stored in the cloud.
from google.colab import drive
drive.mount('/content/drive')
Library Imports and Warning Suppression.
This code imports libraries for data manipulation, visualization, and modeling namely Scikit learn and Statsmodels. It suppresses certain warnings like FutureWarning and ConvergenceWarning to give cleaner output while running.
# Import necessary libraries for data manipulation, visualization, and modeling
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from matplotlib.ticker import FormatStrFormatter
import seaborn as sns
import pylab
import scipy
import warnings
# Suppress specific FutureWarning
warnings.filterwarnings("ignore", category=FutureWarning)
from sklearn.metrics import mean_absolute_error
from sklearn.gaussian_process import GaussianProcessRegressor
from sklearn.gaussian_process.kernels import WhiteKernel, ExpSineSquared, ConstantKernel, RationalQuadratic
from statsmodels.graphics.tsaplots import plot_acf, plot_pacf
# Import ConvergenceWarning from sklearn.exceptions
from sklearn.exceptions import ConvergenceWarning # This line is added
# Suppress ConvergenceWarning messages
warnings.filterwarnings("ignore", category=ConvergenceWarning)
Setting Seaborn Style
This just sets up the color palette for aesthetic plots Seaborn handles the 'white grid' background config.
# Setting Seaborn style for aesthetic plots
sns.set_style("whitegrid")
sns.set_palette("husl")
STEP 2:
Loading Data and Checking Shape
This code loads the CSV file. After loading the dataset, it prints the dataset’s shape to check the number of rows and columns. The %time magic command in the notebook records the time taken to perform the task.
# Load dataset from Google Drive
data_path = "/content/drive/MyDrive/New 90 Projects/Project_12/Data/CallCenterData.xlsx"
raw_data = pd.read_excel(data_path)
print("Dataset Shape:", raw_data.shape)
Previewing Data
This code displays the dataset's first few rows for a quick overview.