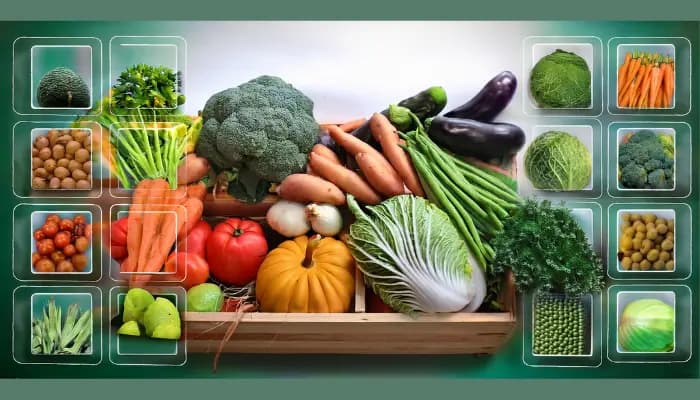
Vegetable classification with Parallel CNN model
The Vegetable Classification project shows how CNNs can sort vegetables efficiently. As industries like agriculture and food retail grow, automating vegetable identification becomes crucial. This project provides a guide to building both custom and parallel CNN models. These models help improve classification accuracy, reduce manual effort, and enhance quality control.
By learning these methods, you can solve harder classification problems in many areas. The project shows how machine learning helps with smart farming, inventory, and sustainability. It highlights how automating vegetable sorting can benefit real-world tasks.
Project Overview
This project aims to build a parallel CNN model that classifies vegetable types. The model analyzes vegetable images and finds key features that set each type apart. It uses two branches of convolutional layers. These branches merge into a dense network for classification. We also trained a traditional CNN model to compare its performance with that of parallel CNN. We check the results to ensure vegetables are sorted correctly into their categories.
This tutorial covers data preparation, model evaluation, and visualization. It also includes troubleshooting steps. Along with vegetable sorting, it teaches key machine learning ideas. These include data cleaning, augmentation, and model improvement. These concepts apply to broader classification tasks as well.
Prerequisites
Before starting, you should know the basics of Python and machine learning. You should also understand deep learning concepts. Specifically, knowledge of convolutional neural networks (CNNs) will be essential. Familiarity with TensorFlow and Keras will make the implementation easier to follow. Experience with datasets and processing image data using Pandas and NumPy is useful.
You need access to Google Colab or Jupyter Notebook to run the code and handle computations. Additionally, Basic knowledge of metrics like accuracy, precision, recall, and AUC is helpful. It will help you measure the model's performance well.
Approach
In this project, we use a machine-learning method with parallel CNN models. The traditional CNN model is our benchmark. The parallel CNN uses multiple branches of layers for deeper feature extraction. We train both models on the same vegetable image dataset. We focus on testing how well the models generalize using validation and test sets.
We use image preprocessing methods like resizing, normalization, and data augmentation. These steps help with training and prevent over-fitting. We also use callbacks like early stopping and learning rate reduction. This improves training time and performance. We show the results with a confusion matrix, classification report, and training metrics.
Workflow and Methodology
The overall workflow of this project includes:
- Data Collection: Gathering images of different vegetable types for classification.
- Data Preprocessing: Resizing, normalizing, and augmenting images to prepare for training.
- Model Design: Building both the custom CNN model and the parallel CNN model.
- Training: Training the models with training data and evaluating them using validation data.
- Evaluation: Testing the models on unseen test data to check classification accuracy.
- Visualization: Plotting metrics like accuracy, precision, recall, AUC, and confusion matrix.
- Optimization: We use callbacks like early stopping and learning rate reduction. These callbacks help improve the model.
The methodology involves:
-
Data Preprocessing: We resize and normalize raw images. This converts them into tensors for CNN input. This ensures the model processes consistent image sizes.
-
CNN Architecture: We design convolutional layers for traditional and parallel CNN models. These layers extract important features for classification.
-
Metrics: We evaluate model performance using accuracy, precision, recall, and AUC. This ensures a thorough performance check.
-
Callbacks: We use callbacks like early stopping and learning rate reduction. These help prevent overfitting and improve training efficiency.
Data Collection
The dataset contains images of vegetables like tomatoes, cucumbers, bean, bitter gourd, brinjal, broccoli, cabbage, capsicum, carrot, cauliflower, papaya, potato, pumpkin and radish.
We divided the dataset into three parts:
- Training.
- Validation, and
- Test sets.
The images are stored in separate directories for easy model training and evaluation. You can upload the dataset to Google Drive and mount it in Google Colab for quick access during the project.
Data Preparation
The images first resize to 224x224 pixels to ensure uniformity in the dataset. Next, we apply normalization to scale pixel values between 0 and 1, which speeds up model training. Additionally, We use data augmentation methods like flipping, rotation, and zooming. This creates more diverse training data. This process reduces overfitting and improves the model's ability to generalize.
Data Preparation Workflow
- Image Resizing: We make sure all images are 224x224 pixels. This keeps the dimensions uniform.
- Normalization: We scale pixel values from 0-255 to 0-1. This improves training efficiency.
- Augmentation: We use data augmentation methods like flipping, rotation, and zooming. This creates more diverse training data.
- Dataset Splitting: We split the dataset of training, validation, and test. This helps us develop and evaluate the model properly.
Code Explanation
STEP 1:
You can mount your Google Drive in a Google Colab notebook with this piece of code. This makes it easy to view files saved in Google Drive. In Colab, you can change and analyze data. You can also train models.
from google.colab import drive
drive.mount('/content/drive')
Import the necessary packages.
This block of code sets up the necessary tools and layers to build a CNN model. It trains and evaluates the model for image classification using TensorFlow and Keras.