- DeepSeek AI and Cerebras: A Game-Changer in AI Infrastructure
- 20 Must-Have Python Libraries for Data Scientists in 2025
- Top 60 Interview Questions for Generative AI
- A Beginner’s Guide to Creating an AI Chatbot
- NextGen AI Voice Assistants
- AI Projects: Beginner to Advanced Levels
- Efficient Text Summarization for Large Documents Using LangChain
- [Solved] 'super' object has no attribute '__sklearn_tags__'
- How to Become a Data Modeler in 2025
- How to Optimize Machine Learning Models with Grid Search in Python
- How to Use the Column Renamed Method in Spark to Rename Columns
- How to Easily Solve Multi-Class Classification Problems in Python
- How to Convert an Image to a Tensor Using PyTorch
- One-Hot Encoding with Multiple Labels in Python
- 10 features engineering techniques for machine learning
- 10 Best LLM Project Ideas to Boost Your AI Skills
- [Solved] OpenAI Python Package Error: 'ChatCompletion' object is not subscriptable
- [Solved] OpenAI API error: "The api_key client option must be set either by passing api_key to the client or by setting the OPENAI_API_KEY env variable"
- Target modules for applying PEFT / LoRA on different models
- how to use a custom embedding model locally on Langchain?
How to Use DeepSeek API for Free: Hands-On Integration Guide
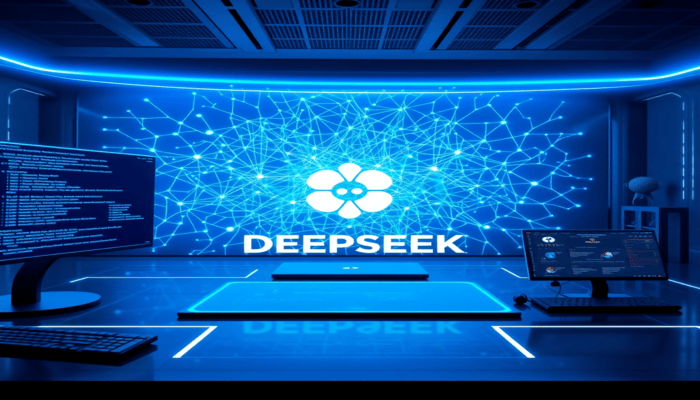
The DeepSeek series of language models, including R1 and V3, have emerged as versatile tools for developers tackling code generation, text analysis, and problem-solving tasks. With Fireworks.ai, a platform offering $1 in free credits, you can experiment with both models at minimal cost. The guide demonstrates methods to obtain free access to DeepSeek R1 and V3 while providing relative feature assessments and implementing AI applications through Python and Google Colab.
Why Fireworks.ai?
Fireworks.ai is a developer-first platform offering:
- Free Tier: $1 credit (enough for 1.1M tokens with R1 or 833K tokens with V3).
- Low-Cost Models: Affordable pricing for both R1 and V3.
- LangChain Integration: Simplify AI workflows with pre-built tools.
- Scalability: Deploy models from prototyping to production.
Step-by-Step Setup
Step 1: Sign Up for Fireworks.ai
- Visit Fireworks.ai and create an account.
- Generate an API Key under API Keys.
Step 2: Set Up Google Colab
- Open a new Colab notebook.
- Install required libraries:
!pip install -qU langchain-fireworks
- langchain-fireworks integrates Fireworks.ai with LangChain, a framework for building AI apps.
- The -qU flag quietly upgrades existing installations.
Step 3: Secure Your API Key
Store your API key in Colab's secrets vault:
- Click the key icon in the left sidebar.
- Add a secret named FIREWORKS_API_KEY and paste your key.
- Load the key into your environment:
from google.colab import userdata
import os
fireworks_api_key = userdata.get('FIREWORKS_API_KEY')
os.environ["FIREWORKS_API_KEY"] = fireworks_api_key
Explanation:
- userdata.get() fetches the secret without exposing it in your code.
- Storing the key in os.environ makes it accessible to the LangChain library.
Step 4: Using DeepSeek R1
- R1 is ideal for quick, straightforward tasks:
from langchain_fireworks import ChatFireworks
llm_r1 = ChatFireworks(
model="accounts/fireworks/models/deepseek-r1",
temperature=0.3,
max_retries=2
)
- model: Unique identifier for DeepSeek R1 on Fireworks.
- temperature (0-1): Controls randomness. Lower = factual, Higher = creative.
- max_retries: Retries failed API calls (e.g., network issues).
Example: Code Debugging
response = llm_r1.invoke("Fix this Python code: print('Hello, World!')")
print(response.content)
Output
# Output: "The code is correct. To add a newline, use print('Hello, World!', end='\n')."
Step 5: Using DeepSeek V3
V3 handles complex reasoning and detailed responses:
llm_v3 = ChatFireworks(
model="accounts/fireworks/models/deepseek-v3", # V3 model ID (confirm on Fireworks)
temperature=0, # Precise answers
max_retries=2
)
Example: Mathematical Reasoning
query = "Solve for x: 2^(x+3) = 32. Explain step-by-step."
response = llm_v3.invoke(query)
print(response.content)
Output:
think>
Okay, so I need to solve the equation 2^(x+3) = 32. Hmm, let's see. I remember that when dealing with exponential equations, the goal is usually to get the variable out of the exponent. One common way to do that is by taking the logarithm of both sides, but maybe there's a simpler way here since both sides are powers of 2? Let me check.
First, let me recall that 32 is a power of 2. Let me think: 2^1 is 2, 2^2 is 4, 2^3 is 8, 2^4 is 16, and 2^5 is 32. Oh, right! So 32 is 2^5. That might make things easier because if I can express both sides of the equation with the same base, then I can set the exponents equal to each other. That's a property of exponents, right? If a^b = a^c, then b = c, as long as a isn't 0 or 1. Since 2 is the base here and it's definitely not 0 or 1, that should work.
So, rewriting the equation: 2^(x+3) = 2^5. Now that both sides have the same base, I can set the exponents equal. That gives me x + 3 = 5. Then, solving for x, I subtract 3 from both sides: x = 5 - 3, so x = 2. Let me check that to make sure I didn't make a mistake.
Plugging x = 2 back into the original equation: 2^(2 + 3) = 2^5 = 32. Yep, that's correct. So x = 2 is the solution. Wait, but just to be thorough, what if I approached this using logarithms instead? Let me try that method to confirm.
Starting with 2^(x+3) = 32. Taking the logarithm base 2 of both sides: log₂(2^(x+3)) = log₂(32). The left side simplifies because log base 2 of 2 to any exponent is just the exponent itself. So that becomes (x + 3) = log₂(32). But log₂(32) is 5, since 2^5 = 32. So again, x + 3 = 5, leading to x = 2. Same result. Good, that confirms it.
Alternatively, if I used natural logarithm or common logarithm, would that work too? Let's see. Using natural logarithm, take ln of both sides: ln(2^(x+3)) = ln(32). Using the logarithm power rule, that becomes (x + 3) * ln(2) = ln(32). Then, solving for x: x + 3 = ln(32)/ln(2). Calculating ln(32)/ln(2), since 32 is 2^5, ln(32) is ln(2^5) = 5*ln(2). So ln(32)/ln(2) = 5*ln(2)/ln(2) = 5. Therefore, x + 3 = 5, so x = 2. Same answer again. So regardless of the method, the solution is x = 2.
I guess the key takeaway here is that if both sides of the equation can be expressed with the same base, it's more straightforward to set the exponents equal. But if not, using logarithms is a reliable method. In this case, since 32 is a power of 2, the first method is simpler. If the equation had different bases that couldn't be easily converted, then logarithms would be the way to go.
Another thing to note is that exponential functions are one-to-one, which means that if two exponential expressions with the same base are equal, their exponents must be equal. That's why setting the exponents equal works here. It's a fundamental property that's really useful for solving these types of equations.
Let me also consider if there are any other possible solutions. Since 2^x is a strictly increasing function, it will only cross any horizontal line once, so there's only one solution. Therefore, x = 2 is the only answer. There's no chance of another solution popping up, unlike in some quadratic or higher-degree equations where multiple solutions might exist.
Just to recap the steps clearly:
1. Recognize that 32 is a power of 2 (2^5).
2. Rewrite the original equation 2^(x+3) = 32 as 2^(x+3) = 2^5.
3. Since the bases are equal, set the exponents equal: x + 3 = 5.
4. Solve for x: x = 5 - 3 = 2.
5. Verify by plugging x = 2 back into the original equation to confirm it holds true.
This problem was a good example of how recognizing common powers can simplify solving exponential equations. If the equation had been something like 3^(x+1) = 27, the same approach would apply since 27 is 3^3. It's all about expressing both sides with the same base whenever possible.
Alternatively, if the equation was 2^(x+3) = 30, which isn't a power of 2, then logarithms would be necessary. In that case, you'd take log base 2 of both sides (or natural log) and solve for x using the logarithm properties. But in this problem, since 32 is a known power of 2, the process is much more straightforward.
I think that's about it. The key steps are recognizing the common base, rewriting the equation, equating the exponents, solving for x, and verifying the solution. This method ensures accuracy and avoids unnecessary complex calculations. It's always good to check the solution by substituting back into the original equation, just to be sure there were no algebraic mistakes made along the way.
</think>
To solve the equation \(2^{x+3} = 32\), follow these steps:
1. **Express 32 as a power of 2**:
Recognize that \(32 = 2^5\). This allows both sides of the equation to have the same base.
2. **Rewrite the equation**:
Substitute \(32\) with \(2^5\):
\[
2^{x+3} = 2^5
\]
3. **Set the exponents equal**:
Since the bases are identical, the exponents must be equal:
\[
x + 3 = 5
\]
4. **Solve for \(x\)**:
Subtract 3 from both sides:
\[
x = 5 - 3 \quad \Rightarrow \quad x = 2
\]
5. **Verify the solution**:
Substitute \(x = 2\) back into the original equation:
\[
2^{2+3} = 2^5 = 32
\]
The equation holds true, confirming the solution.
**Final Answer:**
\(\boxed{2}\)
Step 6: Advanced Use Cases
Case 1: Prompt Templating with LangChain
from langchain.prompts import PromptTemplate
template = """
You are a senior developer. Explain the bug in this code and fix it:
Code: {code}
"""
prompt = PromptTemplate(
template=template,
input_variables=["code"]
)
# Format the prompt
code_snippet = "def add(a, b): return a - b"
_input = prompt.format(code=code_snippet)
# Generate response
response = llm_r1.invoke(_input)
print(response.content)
Output:
Case 2: Comparative Analysis (R1 vs. V3)
Test both models on the same task:
query = "Explain recursion in programming."
# Using R1
response_r1 = llm_r1.invoke(query)
# Using V3
response_v3 = llm_v3.invoke(query)
print("=== R1 Response ===")
print(response_r1.content)
print("\n=== V3 Response ===")
print(response_v3.content)
Output for DeepSeek R1
Output for DeepSeek V3
Using DeepSeek Models
Model Comparison
Model | Best For | Strengths | Weaknesses |
R1 | Basic Q&A, simple tasks | Fast, low-cost | Less context retention |
V3 | Complex reasoning, coding | Higher accuracy | Higher cost per token |
Step 8: Troubleshooting
- Model Not Found: Verify the model ID in the Fireworks Model Dashboard.
- Rate Limits: Fireworks allows 60 requests/minute. Add delays with time.sleep(1) if needed.
- Key Errors: Ensure secrets are named FIREWORKS_API_KEY in Colab.
Conclusion
With DeepSeek R1 and V3 on Fireworks.ai, you can build AI-powered tools for free whether you're debugging code, solving math problems, or generating content. The $1 credit offers ample room for experimentation, and the LangChain integration simplifies development.
Next Steps:
- Explore the Fireworks Documentation.
- Experiment with different temperature settings.
- Monitor usage in the Fireworks Dashboard.
Start coding with this Google Colab Template and unleash the power of AI today!
FAQ
Q: Can I use both models in the same project?
A: Yes! You can initialize R1 and V3 separately and route tasks based on complexity.
Q: How do I check my remaining credits?
A: Visit the Fireworks Usage Dashboard to monitor your available credits.
Q: What happens when I run out of free credits?
A: You can purchase additional credits through Fireworks.ai's pricing plans.
Q: Are these models suitable for commercial use?
A: Review Fireworks.ai's Terms of Service for licensing details regarding commercial applications.
Q: Can I fine-tune DeepSeek models on my data?
A: Fireworks.ai does not currently offer custom fine-tuning for DeepSeek models, but you can use prompt engineering to tailor outputs.